The Phoenix Project
A path in migration!
Legacy COBOL migration to Microsoft Visual Basic .Net (VB.Net)
I was browsing the Internet years ago and came across a paper "The Realities of Language
Conversions" by A.A. Terekhov* and C.
Verhoef explaining the
intricacies of migrating applications from high level languages such as COBOL and
PL/1 to Java or other open languages. For the most part this document points to
straight conversion concepts. In most applications there is no need to convert
all of the code from one language to another.
Primarily, there are 3 areas within a conversion to be concerned with. Each
area has its own specific problems with conversions or "migrations". These
are Screens (User-Interface - UI), Data (Data Access Layer - DAL), and the Business
Logic (Business Logic Layer - BLL). Complete conversion of structured code
from a legacy system to a windows systems is rarely a requirement and unnecessary for most business requirements. The
of parsing code and translating from one language to another is what we did!
Consider the following:
Cobol has a statemen that is used to "START" reading a file
based on either key fields, alternate keys or to start reading from the beginning. The START function
returns an event to signify whether a valid record was read or not (record lock,
found, etc). It does not actually retrieve the record if one was found, but signifies,
based on the event, whether a valid record exists. If a valid record was found,
the READ NEXT function is used to retrieve the record and is repeated until the
EOF (End Of File) event is set which has to be checked for each read. In addition
the number of records found is not available unless you read each record with a
counter and then you have to close the record and restart your START statement.
The amount of code to migrate this code from COBOL to C or VB is unnecessary and
useless. For example, .NET's data layer methods, we obtain the number of rows (records)
found on SqlDataAdapter's Fill method. The START, READ-NEXT (with events) is obsolete,
negating the necessity to migrate any COBOL code which manipulates datafiles with
any of the CRUD functions (Create, Retrieve, Update, Delete).
An Example of structures via VB.Net; autogenerated by the DAL Generator
Public Function isValid_PK_AAJ_REC_AAJ_REC(ByVal AAJ_ASSET_GROUP as Int16, _
ByVal AAJ_ASSET_NUMBER as String) As DataSet
Dim rcnt As Integer = 0
Dim cnstr As String = ConfigurationManager.AppSettings("DBCONN")
Dim sr As SqlConnection = New SqlConnection(cnstr)
Dim sqlcmd As SqlCommand = New SqlCommand
Dim ds As DataSet = New DataSet("AAJ_REC")
Try
sqlcmd.Connection = sr
sqlcmd.CommandText = "spr_PRX_Select_PK_AAJ_RECAAJ_REC
sqlcmd.CommandType = CommandType.StoredProcedure
sqlcmd.Parameters.AddWithValue("@AAJ_ASSET_GROUP", AAJ_ASSET_GROUP)
sqlcmd.Parameters.AddWithValue("@AAJ_ASSET_NUMBER", AAJ_ASSET_NUMBER)
Dim sqlDA As SqlDataAdapter = New SqlDataAdapter(sqlcmd)
sr.Open()
sqlDA.FillSchema(ds, SchemaType.Source, "AAJ_REC")
rcnt = sqlDA.Fill(ds, "AAJ_REC")
sqlDA.Dispose()
sqlDA = Nothing
Return ds
Catch ex As Exception
Finally
If sr.State = ConnectionState.Open Then
sr.Close()
End If
ds.Dispose()
ds = Nothing
sr.Dispose()
sr = Nothing
sqlcmd.Dispose()
sqlcmd = Nothing
End Try
End Function
If the record count is > 0, we have data, and if not, then there
was no data retrieved. Converting all the code from COBOL to a language such as
Visual Basic or even Java would be pointless, so building a converter to migrate
all COBOL code to Visual Basic, C# or Java would have to be highly specific. You
would not want all the code converted "As Is".
Another consideration is whether to migrate with screens and data
"as-is" or enhance the application as you migrate. Our feeling on this
subject is that if the environment is relatively simple with respect to the number
of applications, screens and tables, go ahead and enhance as you migrate. If your
environment has hundreds of applications, screens and many transactions which require
updating records in several tables for each transaction, then migrate first, test,
then enhance. Otherwise you will be complicating the migration process and your
testing phase will become enormous.
First convert legacy code and get it operational so it duplicates
your legacy system, then enhance it.
Moving On..
Screen Conversion
First, what you see on the screen when you run the legacy application
needs to be migrated to a new display format or screen. For example,. the
Cobol Accept-Display statements with screen formatting, or the FMS/DECforms screens
of the VAX/ALPHA era were specific to the environments they were developed in. it
was not possible to move these various and sometimes cumbersome screens to Java
or HTML, or even .NET ASPX forms. Many software systems have numerous screens such
as PRAXA, ASK MAN-MAN, MCBA, MAPICS, and BPICS. The first task is to seamlessly
migrate them "as-is" to the new format and platform. One of the utilities
we developed at Konigi was to actually parse the COBOL screen formats and migrate
them initially to Microsoft Visual Basic .FRM files. When .NET began to evolve,
we identified a more suitable platform which was the dynamic ASPX format. .NET gave
us the ability to have screens with similar structure as that of the 80X24 line
text screen of the legacy systems with the addition of controls, events and everything
else a developer or user could possible need.
After extensive testing we were
able to convert 1000+ screens from the Cobol environment to the ASPX.NET platform
in less then 4 minutes. Much to our surprise, this reduced conversion time for the
screens from 2 man years to 8 days for the coding and testing and 4 minutes to actually
convert them all. The following images are of our convert. This application
parses the program to determine which screen files and tables are opened by the
application. The user simply select the required screen and then select "Buildform".
This create a new window with all the labels and textboxes with the same geometry
as the Cobol screen. The user now has the ability to select table field names and
drag-drop them to the required textboxes know as databinding. The code will automatically
generate a link for the table, fieldname and textbox for each drag-drop sequence.
This procedure requires 1-2 minutes/screen. Upon completion, the user simply select
FormDev to ASPX.NET and the new ASPX form is created.
Initial Selection Screen
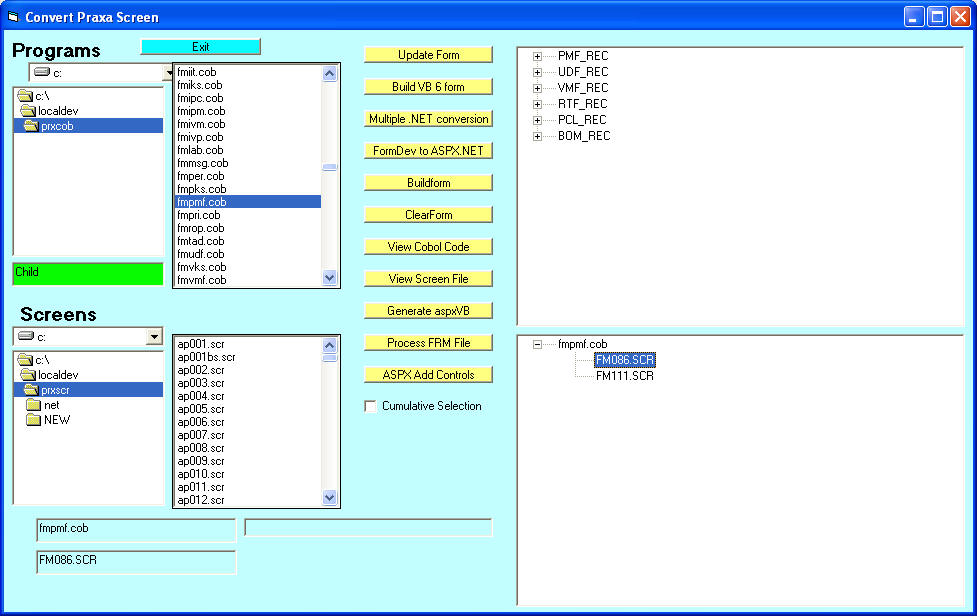
Viewing a Cobol Screen Layout
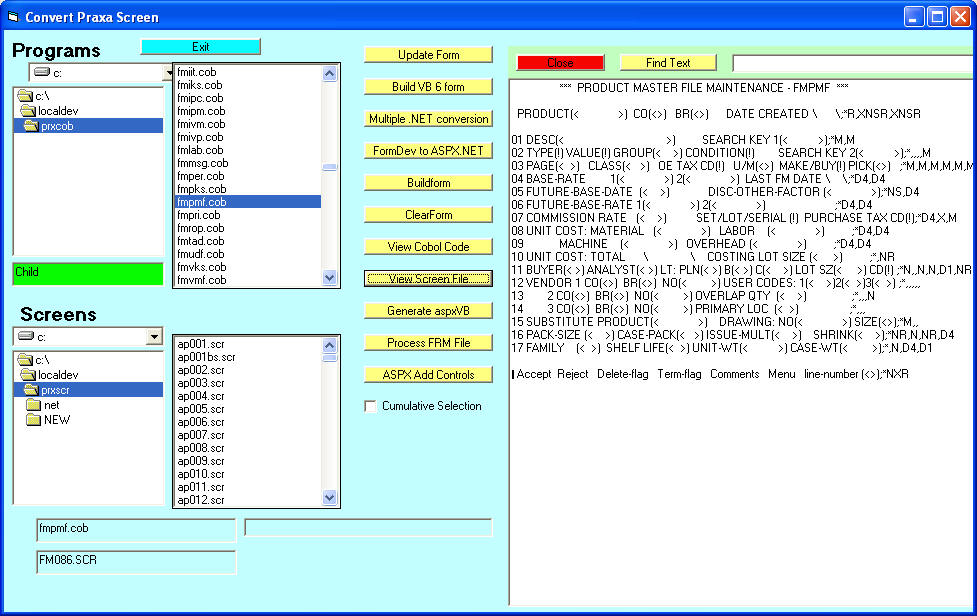
Viewing a converted VB Screen
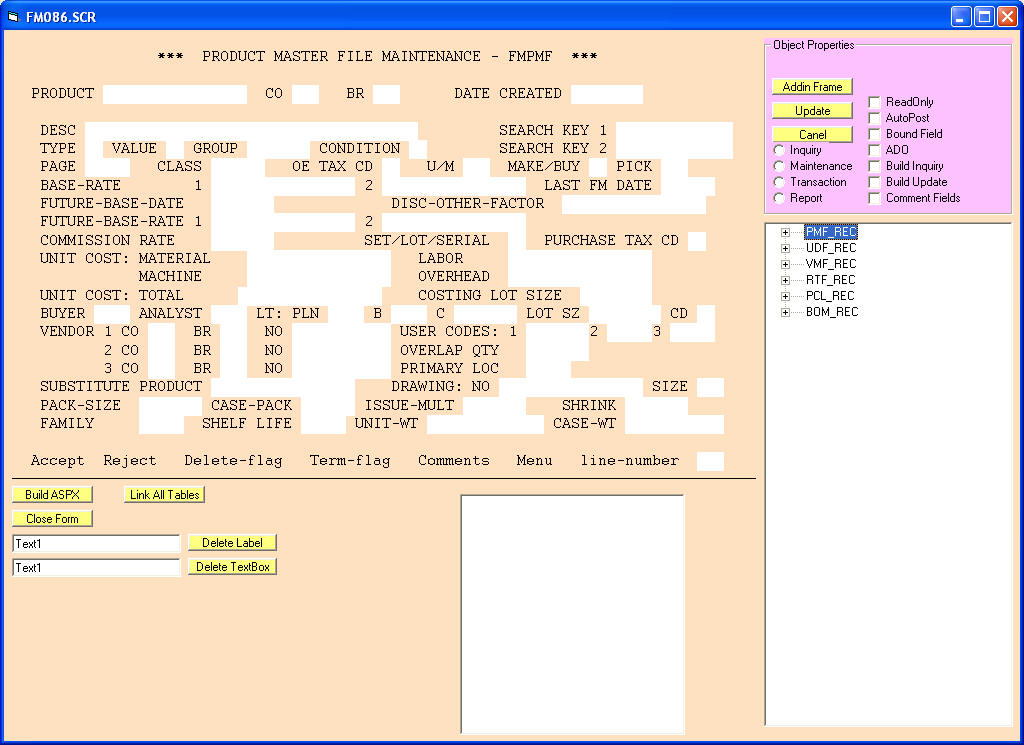
A converted ASPX screen
The final conversion and the one which is vital to us was the binding
of data fields within our SQL tables to our program and converted screens. We designed
an application which allow us to bind the ASPX fields to the SQL table field name
by simply dragging and dropping the field name to the textbox. The environment parses
the COBOL program to determine which data files were used. Using these names, we
used the Schema of the SQL database to present the tables which were used in each
specific program and allow a technician to manually bind fields to controls on the
form. Upon completion, we simply generate the ASPX form with the appropriate controls
bound to SQL tables and fields. A final highlight was to embed the stored procedure
name in a routine in the ASPX program and then link the required stored procedure
fields directly to the form and validate them before executing the stored procedures.
Binding table names to textbox objects.
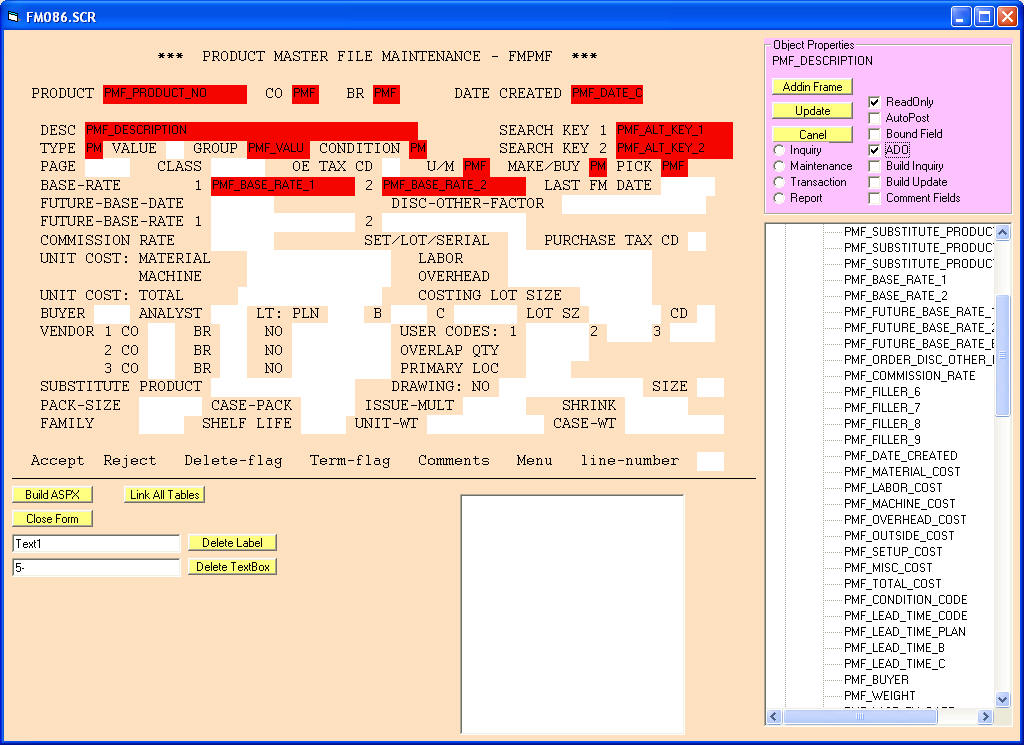
Final Task
The final task is building the functionality for each application,
which is the most time consuming, except with our processes. This requires reading COBOL programs and
translating
the statements, methods functions and calculation in each routine to the the
target language. Most legacy systems
have a large number of queries and reports which do not update data or perform extensive
calculations. These routintes can usually be converted in seconds. The transaction applications are the most difficult to
convert since they my update multiple files (tables) and perform extensive calculations.
The most complex tasks are integrating the Business Logic Layer wtih the
User Interfec. Since many systems have internally designed screen handlers, if
it is difficult to synthesize this linkage. This task is the one element which
requires developers to link Business Logic with Forms.
Testing and debugging converted applications can take days to validate
all the functionality. Using an automatically generated DAL combined with
direct code migration eliminates much fo the Business Logic Testing leaving the
abunace of the testing in the BLL/UI stages.
Conversion of legacy applications to .NET can
be accomplished easily with our processes and tools. The first step is the plan for migration and then the team
to execute the plan. Use the best tools available and the migration will be effective
and fairly painless.
The overwhelming benefit is that you own
the applications, the data and your environment.
For more information on tools and concepts for Legacy code migration,
please contact us at Konigi, Inc..
Richard Ladendecker II
President
Konigi, Inc.
Sales@Konigi.us